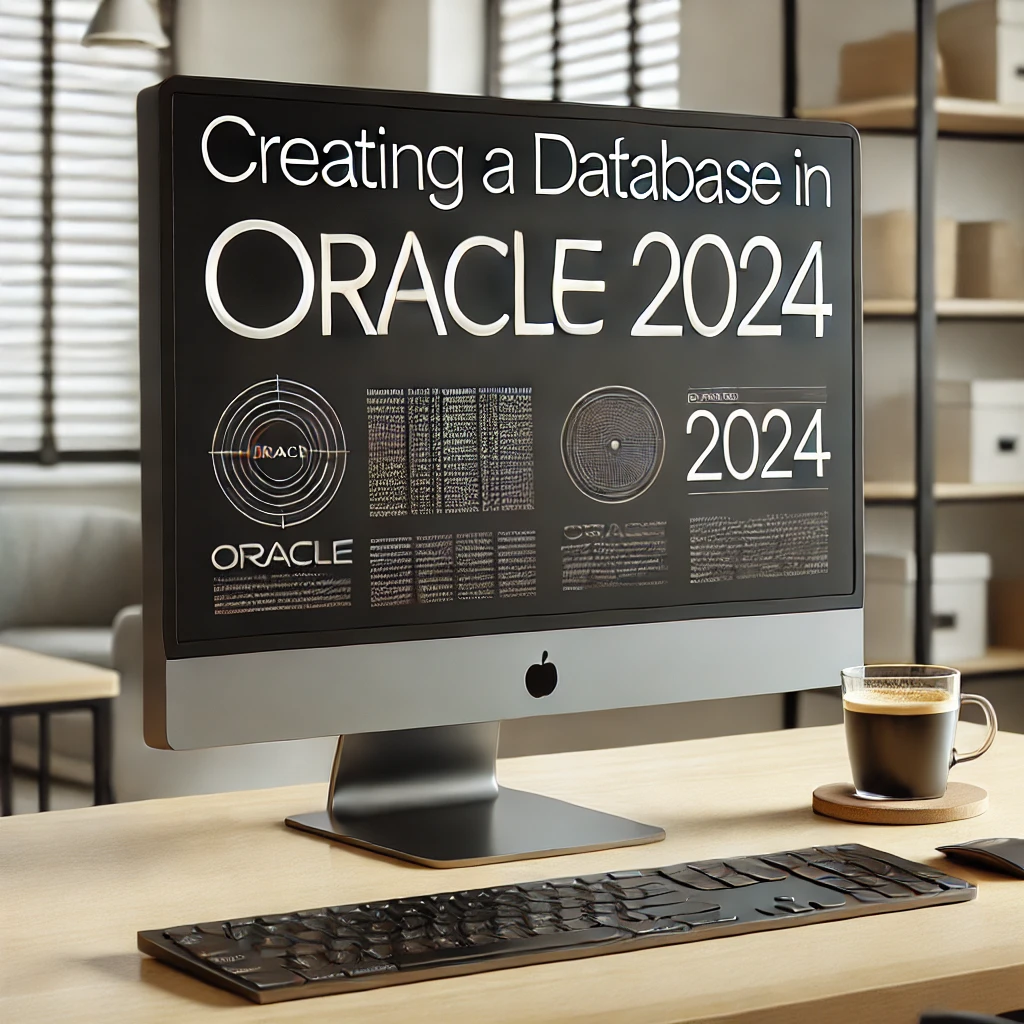
Creating a Database in Oracle 2024
Creating a database in Oracle involves a series of detailed steps that ensure the database is set up correctly for both performance and reliability. This guide will walk you through each part of the process with additional context, best practices, and HTML-formatted code snippets for easy embedding into your blog.
1. Understanding the Basics of Oracle Database Creation
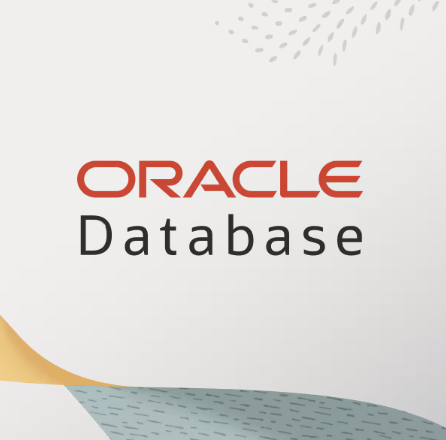
Before diving into database creation, it’s crucial to understand what an Oracle database is and its essential components. An Oracle database consists of a collection of data treated as a single unit. The primary objective of a database is to store and retrieve related information. It is managed by Oracle Database Management System (DBMS) and can be hosted on various platforms.
2. Preparation Steps
Proper planning and preparation before creating a database are essential to avoid performance issues or structural inefficiencies later. Here’s what you need to do:
- Ensure Oracle Software Installation: Confirm that Oracle Database software is installed and configured on your system. Use the Oracle Universal Installer (OUI) to install the software if it isn’t already in place.
- Verify Disk Space: Make sure your system has enough disk space for the datafiles, control files, redo logs, and other components. Insufficient space can cause creation to fail or lead to performance bottlenecks.
- Set Environment Variables: Set the
ORACLE_HOME
andORACLE_SID
environment variables to point to your Oracle installation directory and the desired system identifier (SID).<pre> export ORACLE_HOME=/u01/app/oracle/product/19.0.0/dbhome_1 export ORACLE_SID=myNewDB </pre>
3. Initialization Parameters Setup
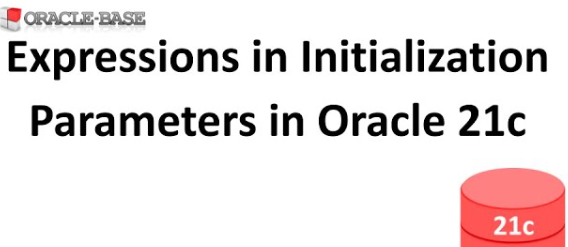
Initialization parameters determine how Oracle will allocate memory, handle processes, and manage other key functions. These can be set in the init.ora
file or through an spfile
. Here are some critical parameters and their implications:
<pre>
<code class="language-sql">
db_name='myNewDB'
control_files=('/u01/app/oracle/oradata/myNewDB/control01.ctl',
'/u01/app/oracle/oradata/myNewDB/control02.ctl')
db_block_size=8192 -- The size of database blocks; must be a multiple of the OS block size
processes=300 -- The maximum number of user processes that can be connected at the same time
sga_target=800M -- Size of the System Global Area (SGA)
pga_aggregate_target=200M -- Size of the Program Global Area (PGA)
undo_management='AUTO'
</code>
</pre>
Best Practices:
db_block_size
: Choose the block size based on your database workload. Larger block sizes can improve performance for data warehousing.sga_target
andpga_aggregate_target
: Allocate sufficient memory to avoid performance degradation, but keep it within the physical RAM available on your system.
4. Creating the Database
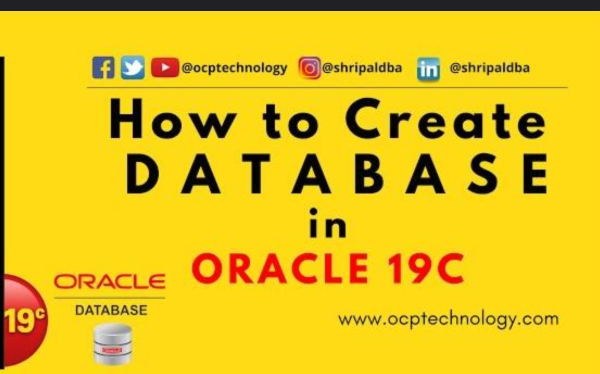
Once the initialization parameters are set, you can proceed with creating the database using the CREATE DATABASE
SQL command. This command defines the database structure and specifies the location of critical files like redo logs, datafiles, and control files.
Comprehensive Example:
<pre>
<code class="language-sql">
CREATE DATABASE myNewDB
USER SYS IDENTIFIED BY "SysPassword"
USER SYSTEM IDENTIFIED BY "SystemPassword"
LOGFILE GROUP 1 ('/u01/app/oracle/oradata/myNewDB/redo01.log') SIZE 100M,
GROUP 2 ('/u01/app/oracle/oradata/myNewDB/redo02.log') SIZE 100M,
GROUP 3 ('/u01/app/oracle/oradata/myNewDB/redo03.log') SIZE 100M
MAXLOGFILES 10
MAXLOGMEMBERS 5
MAXDATAFILES 500
DATAFILE '/u01/app/oracle/oradata/myNewDB/system01.dbf' SIZE 500M AUTOEXTEND ON NEXT 10M MAXSIZE UNLIMITED
SYSAUX DATAFILE '/u01/app/oracle/oradata/myNewDB/sysaux01.dbf' SIZE 100M AUTOEXTEND ON NEXT 10M MAXSIZE UNLIMITED
DEFAULT TEMPORARY TABLESPACE temp TEMPFILE '/u01/app/oracle/oradata/myNewDB/temp01.dbf' SIZE 50M
UNDO TABLESPACE undotbs1 DATAFILE '/u01/app/oracle/oradata/myNewDB/undotbs01.dbf' SIZE 200M AUTOEXTEND ON NEXT 10M MAXSIZE UNLIMITED;
</code>
</pre>
Explanation:
MAXLOGFILES
andMAXLOGMEMBERS
: Define the number of redo log file groups and members per group.AUTOEXTEND
: Enables a datafile to automatically increase in size, reducing the need for manual resizing.SYSAUX Tablespace
: Stores auxiliary database metadata.
5. Creating Control Files
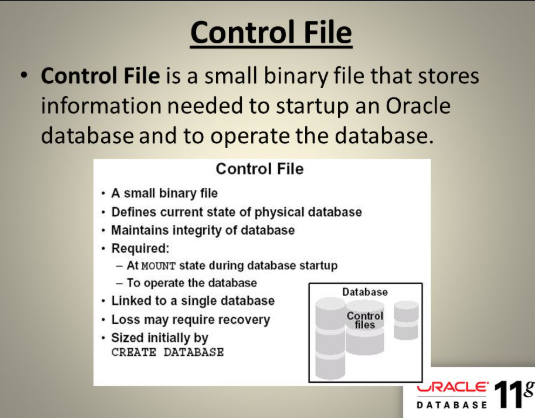
Control files are vital for database functioning. Oracle uses them to track the physical structure of the database. By default, the CREATE DATABASE
command generates them, but it’s recommended to specify multiple control files for redundancy.
<pre>
<code class="language-sql">
ALTER DATABASE BACKUP CONTROLFILE TO TRACE AS '/path/to/control_backup.trc';
</code>
</pre>
This command backs up the control file and generates a trace file that can be used to recreate the control file if needed.
6. Running Post-Creation Scripts
Oracle provides scripts to populate data dictionary views and compile standard packages. These scripts need to be executed immediately after database creation.
<pre>
<code class="language-sql">
@?/rdbms/admin/catalog.sql -- Creates the data dictionary
@?/rdbms/admin/catproc.sql -- Compiles PL/SQL packages and procedures
@?/rdbms/admin/utlrp.sql -- Recompiles any invalid objects
</code>
</pre>
Why These Scripts Are Important:
- The
catalog.sql
script builds the data dictionary, which contains metadata about the database’s structure. - The
catproc.sql
script compiles the standard PL/SQL packages, making them available for use. - The
utlrp.sql
script ensures that all invalid objects in the database are recompiled, preventing runtime errors.
7. Creating Additional Users and Tablespaces
To manage data efficiently, create separate tablespaces and users as needed. This enhances performance and security.
Example of Creating a New Tablespace:
<pre>
<code class="language-sql">
CREATE TABLESPACE myAppData
DATAFILE '/u01/app/oracle/oradata/myNewDB/myAppData01.dbf' SIZE 100M
AUTOEXTEND ON NEXT 10M MAXSIZE UNLIMITED;
</code>
</pre>
Example of Creating a New User:
<pre>
<code class="language-sql">
CREATE USER appUser IDENTIFIED BY "AppPassword"
DEFAULT TABLESPACE myAppData
TEMPORARY TABLESPACE temp
QUOTA UNLIMITED ON myAppData;
</code>
</pre>
Granting Privileges:
<pre>
<code class="language-sql">
GRANT CONNECT, RESOURCE TO appUser;
GRANT CREATE SESSION TO appUser;
</code>
</pre>
8. Database Management and Maintenance
Post-creation, managing your database effectively is crucial for smooth operation. Tasks include:
- Monitoring Performance: Use Oracle tools like AWR (Automatic Workload Repository) and ADDM (Automatic Database Diagnostic Monitor) to analyze performance.
- Backups: Regularly back up your database using RMAN (Recovery Manager) to safeguard against data loss. Example RMAN Command:
<pre>
<code class="language-sql">
RMAN> BACKUP DATABASE PLUS ARCHIVELOG;
</code>
</pre>
9. Validation and Troubleshooting
Once the database is created, verify its status:
<pre>
<code class="language-sql">
SELECT instance_name, status FROM v$instance;
SELECT open_mode FROM v$database;
</code>
</pre>
If the status reads OPEN
and the instance is READ WRITE
, your database is correctly configured.
Best Practices for Database Creation
- Use Multiple Redo Log Groups: This ensures data integrity and minimizes data loss in case of failure.
- Enable ARCHIVELOG Mode: To recover data in the event of a crash, use
ARCHIVELOG
mode.
<pre>
<code class="language-sql">
ALTER DATABASE ARCHIVELOG;
ALTER DATABASE OPEN;
</code>
</pre>
- Document Your Steps: Maintain a record of each step, including parameter values and paths, to make troubleshooting easier.
Conclusion
Creating a database in Oracle involves more than just running a simple command. It requires preparation, knowledge of initialization parameters, and careful execution of SQL commands. This guide ensures that you can create a fully functional Oracle database with all necessary components and best practices, facilitating efficient and secure data management.
This detailed content will ensure a comprehensive understanding of Oracle database creation, enabling readers to apply these practices to real-world scenarios.